We can handle Date data type in Salesforce Agentforce.
In this Blog Post, I have gathered start and end dates from the customer to fetch and display available Products to book opportunity using Salesforce Agentforce.
Sample Apex Code with Dates entered by the user:
public class ProductsAvailabilityCheck {
public class ProductsAvailabilityInput {
@InvocableVariable( required = true )
public Date startDate;
@InvocableVariable( required = true )
public Date endDate;
}
public class ProductsAvailabilityOutput {
@InvocableVariable
public String strmessage;
@InvocableVariable
public List < Product2 > listProducts;
}
@InvocableMethod(
label = 'Fetch Available Products'
description = 'Fetching Available products for the date range'
)
public static List < ProductsAvailabilityOutput > fetchAvailableProducts(
List < ProductsAvailabilityInput > productsAvailabilityInputs
) {
ProductsAvailabilityOutput objOutput = new ProductsAvailabilityOutput();
ProductsAvailabilityInput objInput = productsAvailabilityInputs.get( 0 );
Date endDate = objInput.endDate;
Date startDate = objInput.startDate;
Map < Id, Product2 > mapProducts = new Map < Id, Product2 >( [
SELECT Id, Name
FROM Product2
WHERE IsActive = true
ORDER BY Name
] );
for (
OpportunityLineItem objOLI : [
SELECT Product2Id
FROM OpportunityLineItem WHERE
Product2Id IN: mapProducts.keySet() AND (
( Start_Date__c >=: startDate AND Start_Date__c <=: endDate ) OR
( End_Date__c >=: startDate and End_Date__c <=: endDate ) OR
( Start_Date__c <=: startDate AND End_Date__c >=: endDate )
)
]
) {
mapProducts.remove( objOLI.Product2Id );
}
if ( mapProducts.size() > 0 ) {
objOutput.strmessage = 'Products are available during ' +
String.valueOf( startDate ) + ' and ' + String.valueOf( endDate );
if ( mapProducts.values().size() <= 50 ) {
objOutput.listProducts = mapProducts.values();
} else {
Integer intCounter = 0;
List < Product2 > listProducts = new List < Product2 >();
List < Product2 > tempListProducts = mapProducts.values();
while ( intCounter < 50 ) {
listProducts.add(
tempListProducts.get( intCounter )
);
intCounter += 1;
}
objOutput.listProducts = listProducts;
}
} else {
objOutput.strmessage = 'Products are unavailable during ' +
String.valueOf( startDate ) + ' and ' + String.valueOf( endDate );
}
return new List < ProductsAvailabilityOutput > {
objOutput
};
}
}
Topic Configuration:
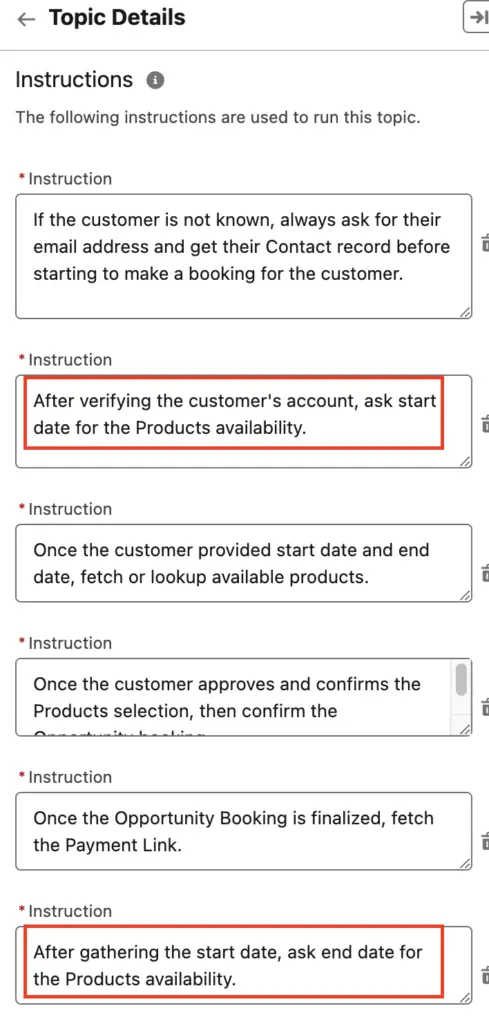
Agent Action Configuration:
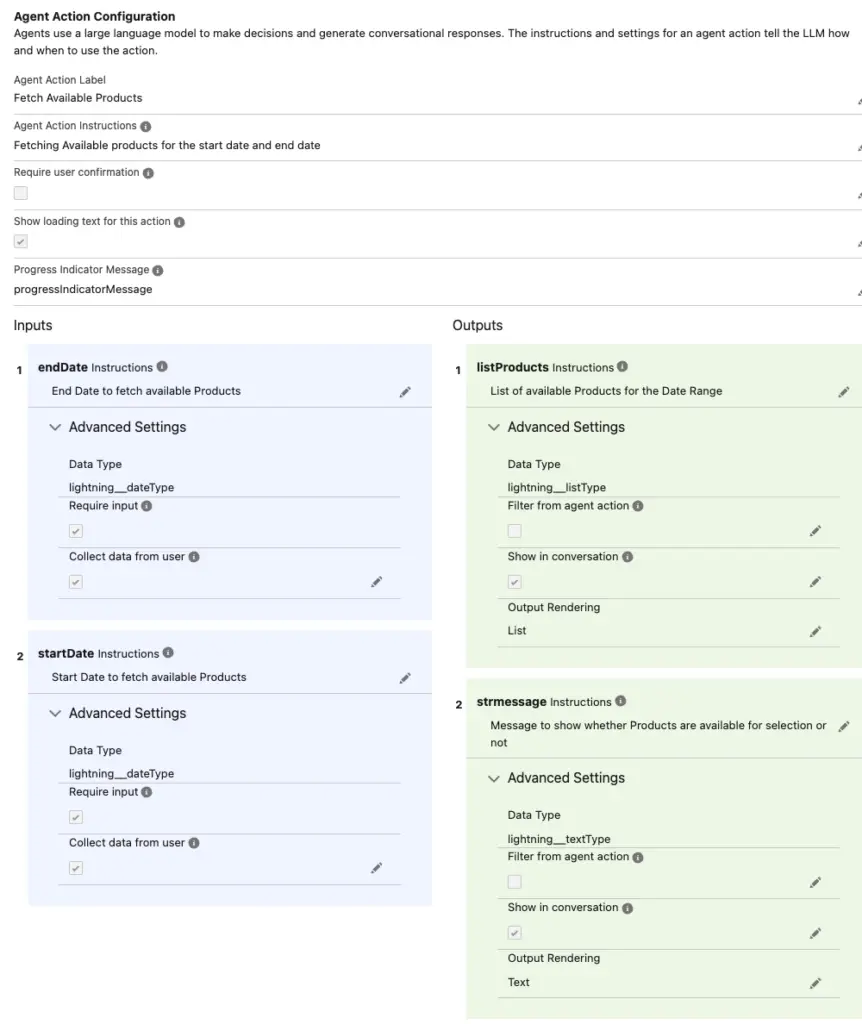
Output:
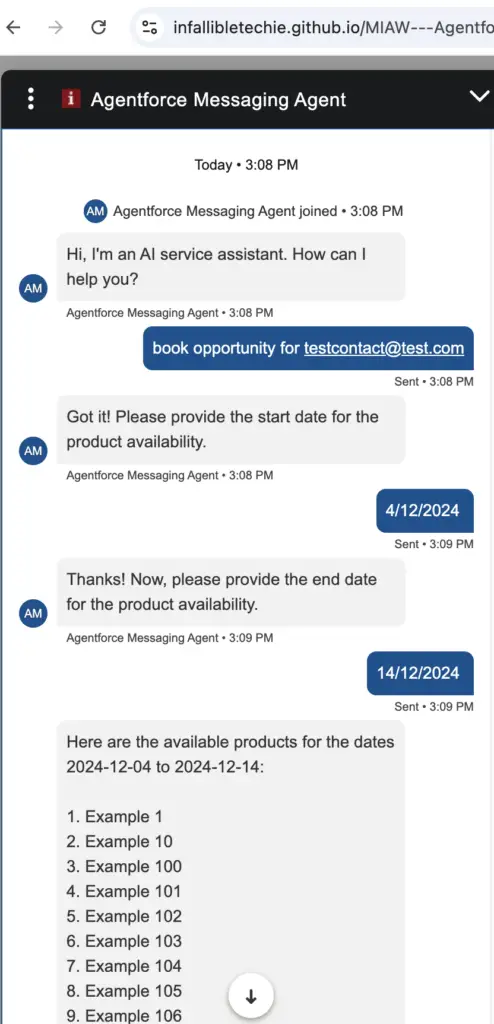