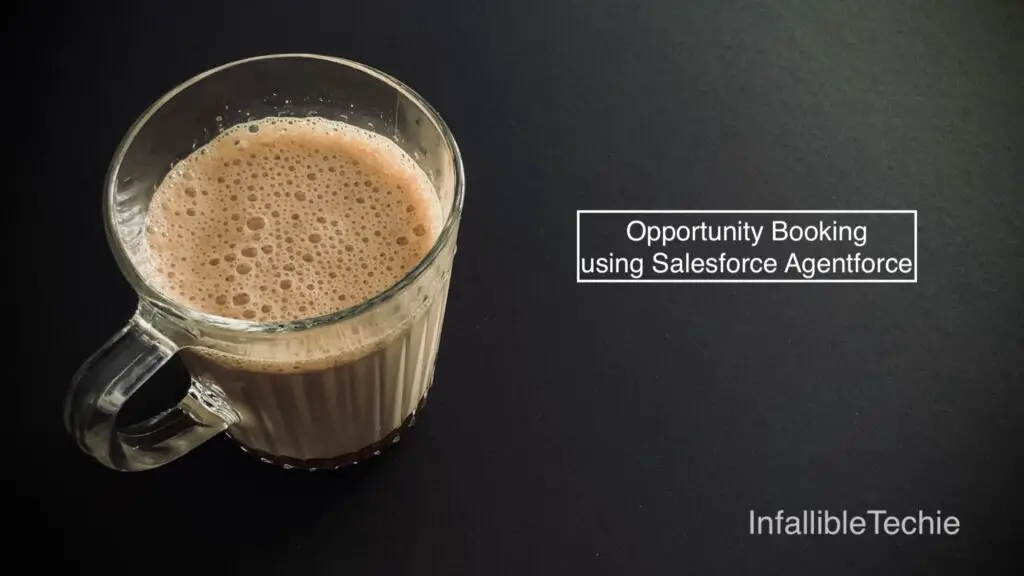
We can handle Opportunity and Opportunity Product creations using the Salesforce Agentforce. In this Blog Post, I have used Apex Agent Action to handle the record creations.
To setup Messaging for In-App and Web, please check
To invoke the Agentforce agent from the Salesforce Messaging for In-App and Web, check the following.
For invoking Apex Agent action, check the following.
Sample Apex Class for Agent Action:
public without sharing class AgentforceFinalizeOrder {
public class FinalizeOrderInput {
@InvocableVariable( required = true )
public String productCity;
@InvocableVariable( required = true )
public Contact objContact;
@InvocableVariable( required = true )
public List < Product2 > listProducts;
}
@InvocableMethod(
label = 'Finalize Order Booking'
description = 'Creating Opportunity and Products to finalize booking'
)
public static List < String > createOpptyAndProducts(
List < FinalizeOrderInput > listInputs
) {
String strResponse = 'Order Booking completed successfully';
List < OpportunityLineItem > listOpptyProducts = new List < OpportunityLineItem >();
Map < Id, PriceBookEntry > mapProductIdPBE = new Map < Id, PriceBookEntry >();
FinalizeOrderInput objInput = listInputs.get( 0 );
String strCity = objInput.productCity;
Contact objContact = objInput.objContact;
List < Product2 > listProducts = objInput.listProducts;
try {
Opportunity objOpportunity = new Opportunity();
objOpportunity.Name = 'Test Agentforce - ' + strCity;
objOpportunity.CloseDate = Date.today();
objOpportunity.ContactId = objContact.Id;
objOpportunity.StageName = 'Prospecting';
insert objOpportunity;
for ( PriceBookEntry objPBE : [
SELECT Id, Product2Id, UnitPrice
FROM PriceBookEntry
WHERE Product2Id IN: listProducts
] ) {
mapProductIdPBE.put(
objPBE.Product2Id,
objPBE
);
}
for ( Product2 objProduct : listProducts ) {
OpportunityLineItem objOLI = new OpportunityLineItem();
objOLI.Quantity = 1;
objOLI.Product2Id = objProduct.Id;
objOLI.OpportunityId = objOpportunity.Id;
objOLI.TotalPrice = mapProductIdPBE.get( objProduct.Id ).UnitPrice;
objOLI.PriceBookEntryId = mapProductIdPBE.get( objProduct.Id).Id;
listOpptyProducts.add( objOLI );
}
insert listOpptyProducts;
} catch ( DMLException e ) {
strResponse = e.getMessage();
}
List < String > returnResponse = new List < String > {
strResponse
};
return returnResponse;
}
}
Test Class:
@isTest
private class TestAgentforceFinalizeOrder {
static testMethod void testFinalizeOrder() {
Contact objContact = new Contact();
objContact.LastName = 'Testing';
insert objContact;
Product2 objProduct = new Product2();
objProduct.Name = 'Test Product';
objProduct.ProductCode = 'TestProduct';
objProduct.IsActive = true;
insert objProduct;
PriceBookEntry objPBE = new PriceBookEntry();
objPBE.UnitPrice = 100;
objPBE.IsActive = true;
objPBE.Product2Id = objProduct.Id;
objPBE.PriceBook2Id = Test.getStandardPricebookId();
insert objPBE;
AgentforceFinalizeOrder.FinalizeOrderInput objInput
= new AgentforceFinalizeOrder.FinalizeOrderInput();
objInput.listProducts = new List < Product2 > {
objProduct
};
objInput.productCity = 'Miami';
objInput.objContact = objContact;
AgentforceFinalizeOrder.createOpptyAndProducts(
new List < AgentforceFinalizeOrder.FinalizeOrderInput > {
objInput
}
);
}
}
Agentforce Configuration:
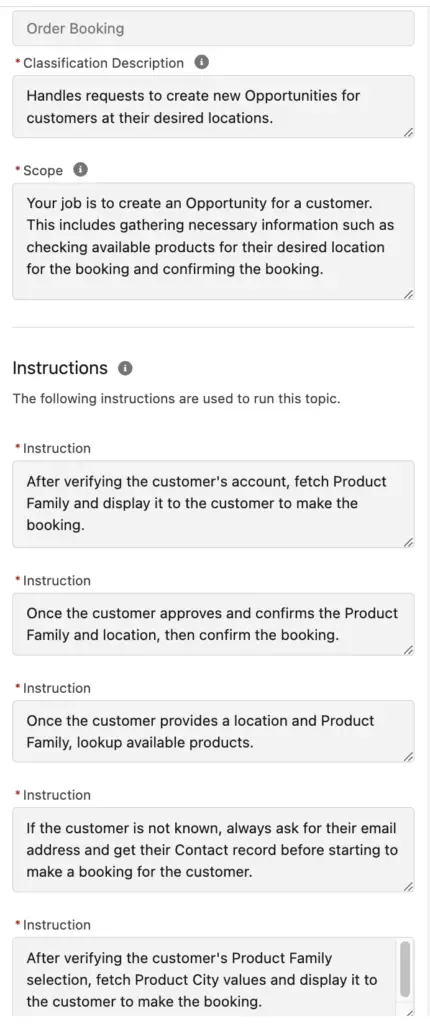
Classification Description
Handles requests to create new Opportunities for customers at their desired locations.
Scope
Your job is to create an Opportunity for a customer. This includes gathering necessary information such as checking available products for their desired location for the booking and confirming the booking.
Instructions
The following instructions are used to run this topic.
After verifying the customer's account, fetch Product Family and display it to the customer to make the booking.
Once the customer approves and confirms the Product Family and location, then confirm the booking.
Once the customer provides a location and Product Family, lookup available products.
If the customer is not known, always ask for their email address and get their Contact record before starting to make a booking for the customer.
After verifying the customer's Product Family selection, fetch Product City values and display it to the customer to make the booking.
Agent Action Configuration:
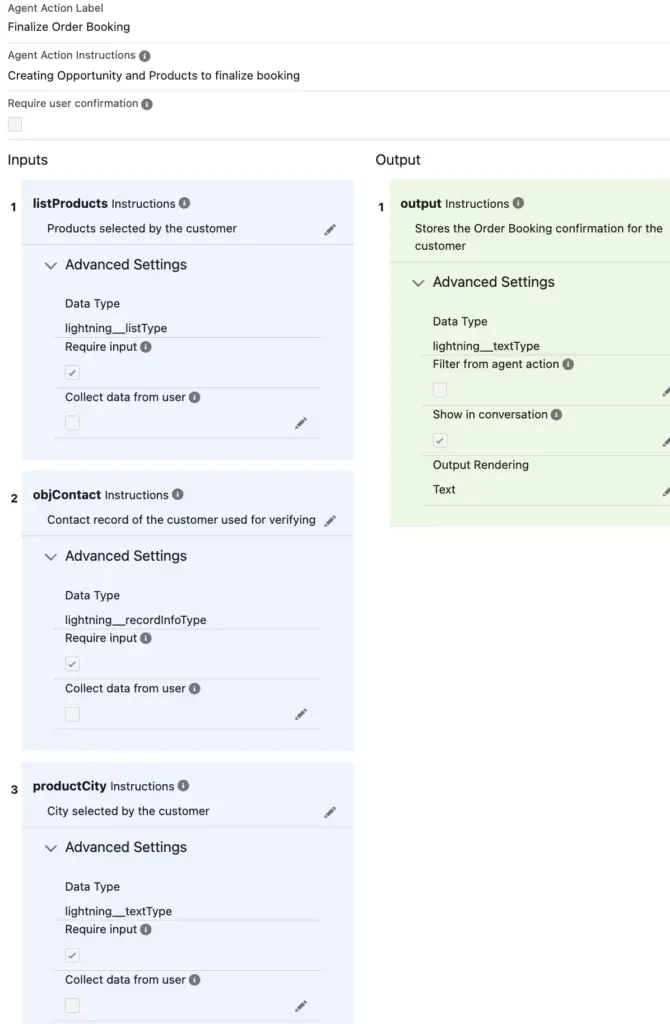