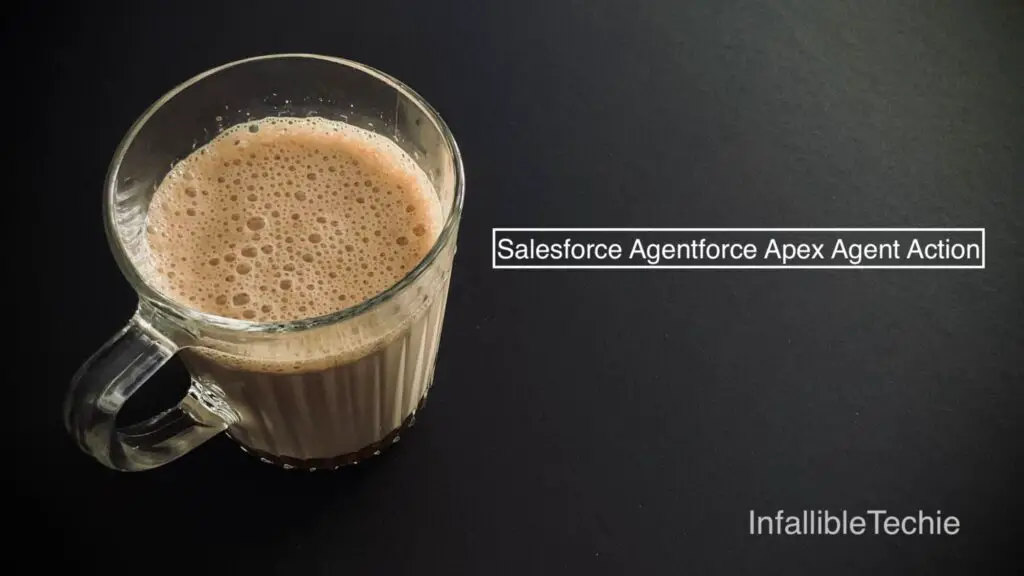
@InvocableMethod annotation helps to invoke apex class and method from the Salesforce Agentforce Apex Action.
@InvocableVariable annotation can be used to pass multiple parameters from the Salesforce Agent Action to the Apex Class.
Sample Apex Class:
public class AgentforceUtility {
public class PicklistInput {
@InvocableVariable( required = true )
public String objectName;
@InvocableVariable( required = true )
public String fieldName;
}
@InvocableMethod(
label='Fetch Picklist Values'
description='Fetching Picklist values for the object and field passed'
)
public static List < List < String > > fetchPicklistValues(
List < PicklistInput > picklistInputs
) {
PicklistInput objPicklistInput = picklistInputs.get( 0 );
String strObjectName = objPicklistInput.objectName;
String strFieldName = objPicklistInput.fieldName;
SObjectType objType
= Schema.getGlobalDescribe().get( strObjectName );
Map < String, Schema.SObjectField > mapFields
= objType.getDescribe().fields.getMap();
SObjectField objField = mapFields.get( strFieldName );
Schema.DescribeFieldResult fieldResult = objField.getDescribe();
List < Schema.PicklistEntry > picklistEntries = fieldResult.getPicklistValues();
List < String > tempProdFamilyPicklistValues = new List < String >();
List < List < String > > productFamilyPicklistValues = new List < List < String > >();
for ( Schema.PicklistEntry objEntry : picklistEntries ) {
tempProdFamilyPicklistValues.add(
objEntry.getValue()
);
}
productFamilyPicklistValues.add(
tempProdFamilyPicklistValues
);
return productFamilyPicklistValues;
}
}
Agentforce Apex Agent Action Configuration:
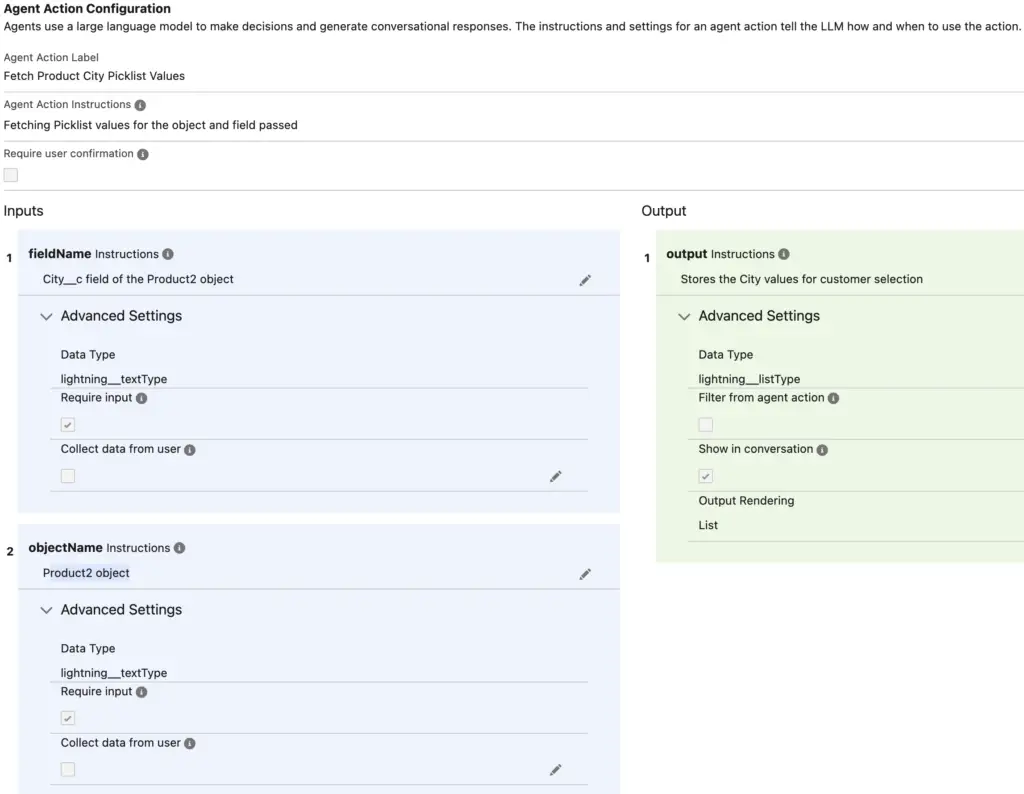
Apex Test class:
@isTest
private class TestAgentforceUtility {
static testMethod void testFetchPicklist() {
AgentforceUtility.PicklistInput objInput
= new AgentforceUtility.PicklistInput();
objInput.objectName = 'Product2';
objInput.fieldName = 'Family';
AgentforceUtility.fetchPicklistValues(
new List < AgentforceUtility.PicklistInput > {
objInput
}
);
}
}