Sample Code:
index.html:
<!DOCTYPE html>
<html lang="en">
<head>
<title>Demo</title>
<!-- Recommended meta tags -->
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width,initial-scale=1.0">
<!-- PyScript CSS -->
<link rel="stylesheet" href="https://pyscript.net/releases/2025.2.1/core.css">
<!-- This script tag bootstraps PyScript -->
<script type="module" src="https://pyscript.net/releases/2025.2.1/core.js"></script>
<style>
.container {
background-color: #f5f5f5;
border-radius: 8px;
padding: 20px;
box-shadow: 0 2px 4px rgba(0,0,0,0.1);
}
#message-list {
margin-top: 20px;
border: 1px solid #ddd;
border-radius: 4px;
padding: 10px;
background-color: white;
height: 150px;
overflow-x: hidden;
overflow-y: auto;
}
</style>
</head>
<body>
<div style="background-color: #000000; color: #1fb5a3; text-align: center; font-weight: bold; font-size:55px;">
Demo
</div>
<br/>
<div class="container">
<h1>Demo Message List</h1>
<div id="message-list"></div>
<br/><br/>
<div>
<input type="text" id="message-input" placeholder="Type your message here...">
<button id="add-btn" py-click="send_message">Send Message</button>
</div>
</div>
<script type="py" src="./main.py" config="./pyscript.toml"></script>
</body>
</html>
main.py:
import datetime
from js import document
# Initialize variables
messages = []
# Send message
async def send_message(event):
now = datetime.datetime.now().strftime( "%Y-%m-%d %H:%M:%S" )
input_element = document.getElementById( "message-input" )
message_text = input_element.value.strip()
if message_text:
messages.append( f"You: {message_text}" )
messages.append( now )
messages.append( "<br/>" )
input_element.value = ""
update_display()
# Update display
def update_display():
message_list = document.getElementById( "message-list" )
html_content = "".join( f'<div class="message-item">{msg}</div>' for msg in messages )
message_list.innerHTML = html_content
document.getElementById( "message-input" ).focus()
pyscript.toml:
name = "Demo"
Output:
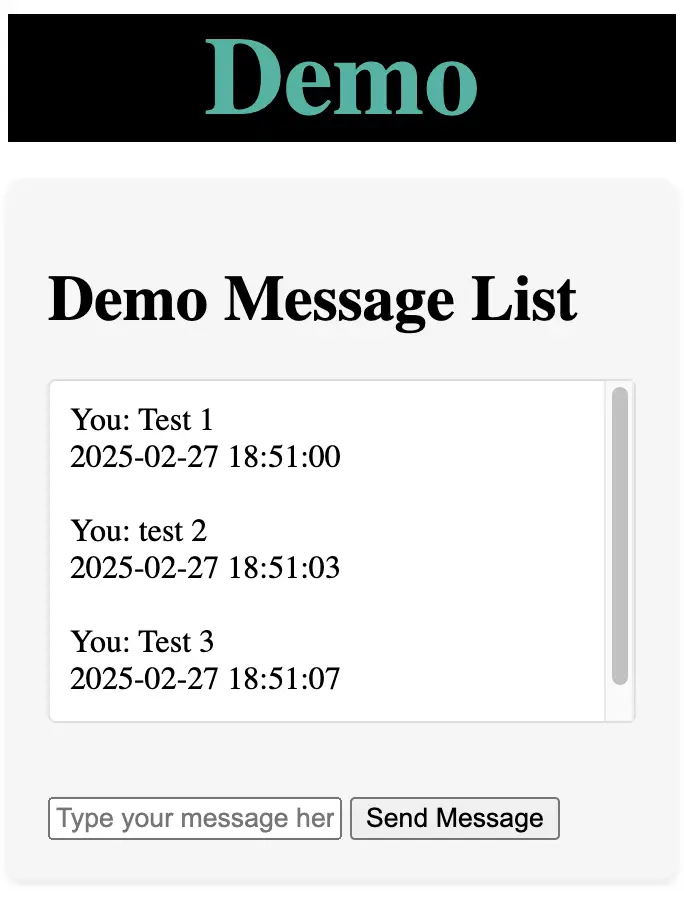