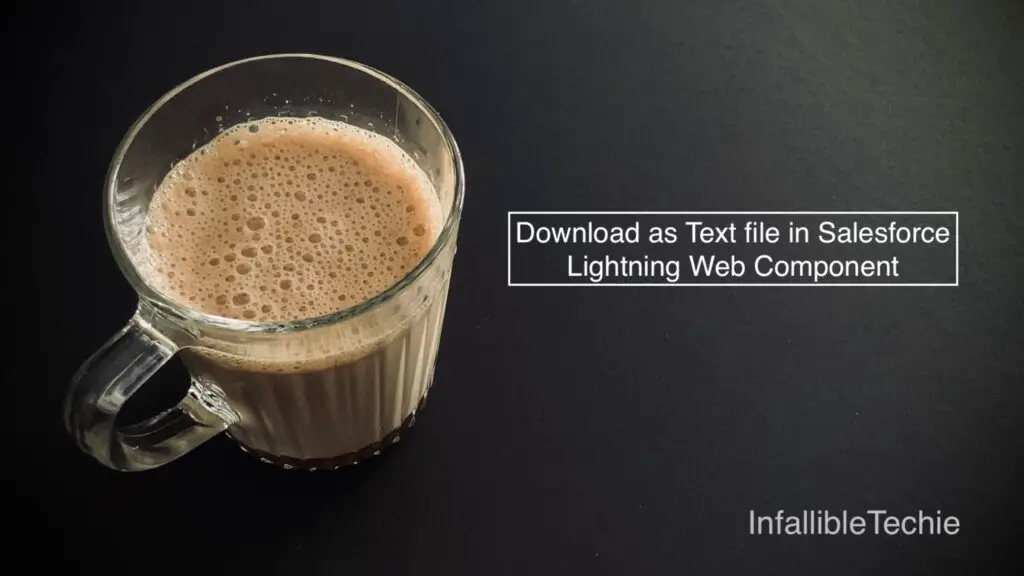
We can download the content as text file in Salesforce Lightning Web Component by creating an anchor element and making use of the click() method.
In this following sample Lightning Web Component, we are downloading the content entered in the text area field.
Sample Lightning Web Component:
HTML:
<template>
<lightning-card>
<lightning-button-icon
slot="actions"
icon-name="utility:download"
alternative-text="Download Conversation"
onclick={handleDownload}>
</lightning-button-icon>
<div class="slds-p-around_medium">
<lightning-textarea
type="text"
title="Message"
label="Message"
value={strMessage}
onchange={handleMessageChange}>
</lightning-textarea>
</div>
</lightning-card>
</template>
JavaScript:
import { LightningElement } from 'lwc';
export default class SampleLightningWebComponent extends LightningElement {
strMessage;
/*
* This method is called when the message is changed in the textarea
*/
handleMessageChange( event ) {
this.strMessage = event.detail.value;
}
/*
* This method is called when Download button is clicked
*/
handleDownload() {
console.log( 'Download Start' );
this.downloadString( this.strMessage, "text/plain", "Testing.txt" );
console.log( 'Download End' );
}
/*
* This method is called to download the string
*/
downloadString( text, fileType, fileName ) {
let objBlob = new Blob( [ text ], { type: fileType } );
let objAnchor = document.createElement( 'a' );
objAnchor.download = fileName;
objAnchor.href = URL.createObjectURL( objBlob );
objAnchor.dataset.downloadurl = [ fileType, objAnchor.download, objAnchor.href ].join( ':' );
objAnchor.style.display = "none";
objAnchor.click();
setTimeout(
function() {
console.log( 'Inside Revoke' );
URL.revokeObjectURL( objAnchor.href );
}, 1000
);
}
}
js-meta.xml:
<?xml version="1.0" encoding="UTF-8"?>
<LightningComponentBundle xmlns="http://soap.sforce.com/2006/04/metadata">
<apiVersion>63.0</apiVersion>
<isExposed>true</isExposed>
<targets>
<target>lightning__Tab</target>
</targets>
</LightningComponentBundle>