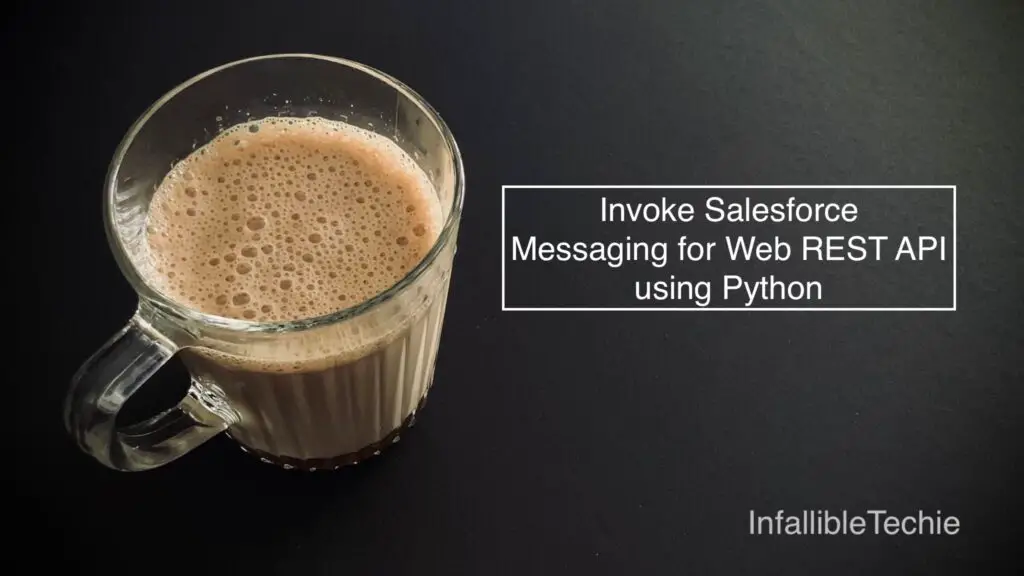
Salesforce Messaging for Web REST API helps us to connect to the Messaging Services via REST API. Check the following link for getting started with the Salesforce Messaging for Web REST API.
In order to do REST API Calls, we need requests library. So, install it using the following command.
python3 -m pip install requests
In order to subscribe to the Server-Side Events from Salesforce, we need sseclient. So, install it using the following command.
python3 -m pip install sseclient
1. Create a Folder to create and run all the Python files. I have used the folder name as “Salesforce MIAW“.
2. Create a Variables.py file. This file will be used to store and handle re-usable variables.
#Conversation Id
conversation_id = ''
#Access Token
access_token = ''
# Messaging for Web baAPIse End Point
base_scrt2_url = 'https://infallibletechiemiaworg.my.salesforce-scrt.com/iamessage/api/v2/'
3. Create a file using the following sample code. This code is used to generate the access token from the Salesforce Messaging for Web REST API.
import requests
import Variables
# POST request to get the Access Token
response = requests.post( Variables.base_scrt2_url + 'authorization/unauthenticated/access-token', json = {
"orgId": "00Dau000002ItPt",
"esDeveloperName": "MIAW_REST_API",
"capabilitiesVersion": "1", "platform":
"Web"
} )
# Retrieving the Access Token
json_res = response.json()
access_token = json_res[ 'accessToken' ]
print(
'Access Token is ' +
access_token
)
# Modifying the Access Token in the Variables.py file
var_name = 'access_token'
file_path = 'Salesforce MIAW/Variables.py';
# Reading the file content
with open( file_path, 'r' ) as file:
lines = file.readlines()
# Modifying the variable
with open( file_path, "w" ) as file:
for line in lines:
if line.startswith( var_name ):
file.write( var_name + ' = \'' + access_token + '\'\n' )
else:
file.write( line )
4. Create a file using the following sample code. This code is used to create the Conversation.
import uuid
import requests
import Variables
conversation_id = str( uuid.uuid4() )
print( conversation_id )
# Modifying the Conversation Id in the Variables.py file
var_name = 'conversation_id'
file_path = 'Salesforce MIAW/Variables.py';
# Reading the file content
with open( file_path, 'r' ) as file:
lines = file.readlines()
# Modifying the variable
with open( file_path, "w" ) as file:
for line in lines:
if line.startswith( var_name ):
file.write( var_name + ' = \'' + conversation_id + '\'\n' )
else:
file.write( line )
# POST request to create the Conversation
response = requests.post(
Variables.base_scrt2_url + 'conversation',
json = {
"conversationId": conversation_id,
"esDeveloperName": "MIAW_REST_API",
"routingAttributes": {
"_firstName": "Test",
"_lastName": "Contact",
"_email": "[email protected]"
}
}, headers={
'Authorization': 'Bearer ' + Variables.access_token
}
)
# Printing the Status Code
print( response.status_code )
5. Create a file using the following sample code. This code is used to listen to the Salesforce Messaging Server-Side Events(SSE).
import Variables
from sseclient import SSEClient
messages = SSEClient(
'https://infallibletechiemiaworg.my.salesforce-scrt.com/eventrouter/v1/sse',
headers = {
'Authorization': 'Bearer ' + Variables.access_token,
'X-Org-Id': '00Dau000002ItPt',
'Accept': '*/*',
'Connection': 'keep-alive',
'Accept-Encoding': 'gzip, deflate, br',
'User-Agent': 'python-requests/2.26.0',
'Host': 'localhost:8080'
}
)
try:
for msg in messages:
print( 'Event: ' + msg.event )
print( 'Data: ' + str( msg.data ) )
print( 'Id: ' + str( msg.id ) )
print( '---------------------' )
except KeyboardInterrupt:
print( "Stream closed by user." )
except Exception as e:
print( f'Error while streaming events: { e }' )
finally:
print( 'Closing the Stream' );
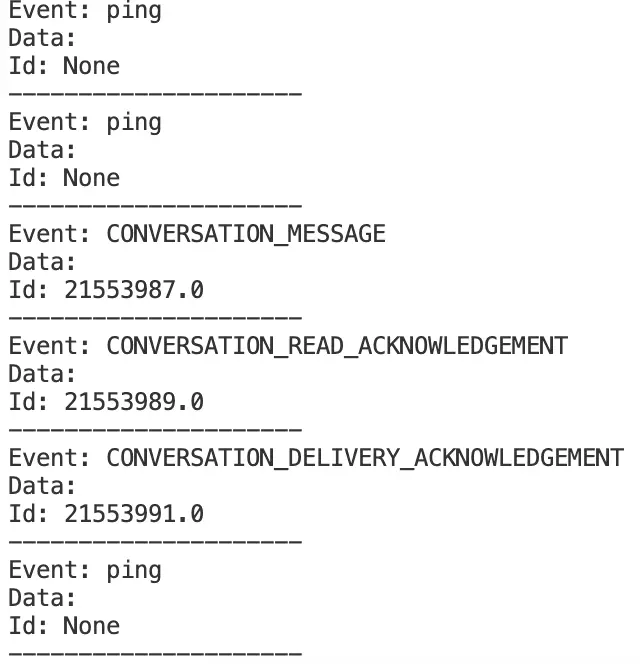
6. Create a file using the following sample code. This code is used to send a message.
import uuid
import requests
import Variables
# POST request to send the Message
response = requests.post(
Variables.base_scrt2_url + 'conversation/' + Variables.conversation_id + '/message',
json = {
"message" : {
"inReplyToMessageId": "",
"id": str( uuid.uuid4() ),
"messageType": "StaticContentMessage",
"staticContent": {
"formatType": "Text",
"text": "Testing from Python"
}
},
"esDeveloperName": "MIAW_REST_API",
"isNewMessagingSession": False,
"routingAttributes": {},
"language": "en"
}, headers={
'Authorization': 'Bearer ' + Variables.access_token
}
)
# Printing the Status Code
print( response.status_code )
7. Create a file using the following sample code. This code is used to close the Conversation.
import requests
import Variables
# POST request to create the Conversation
response = requests.delete(
Variables.base_scrt2_url + 'conversation/' + Variables.conversation_id + '?esDeveloperName=MIAW_REST_API',
headers = {
'Authorization': 'Bearer ' + Variables.access_token
}
)
# Printing the Status Code
print( response.status_code )