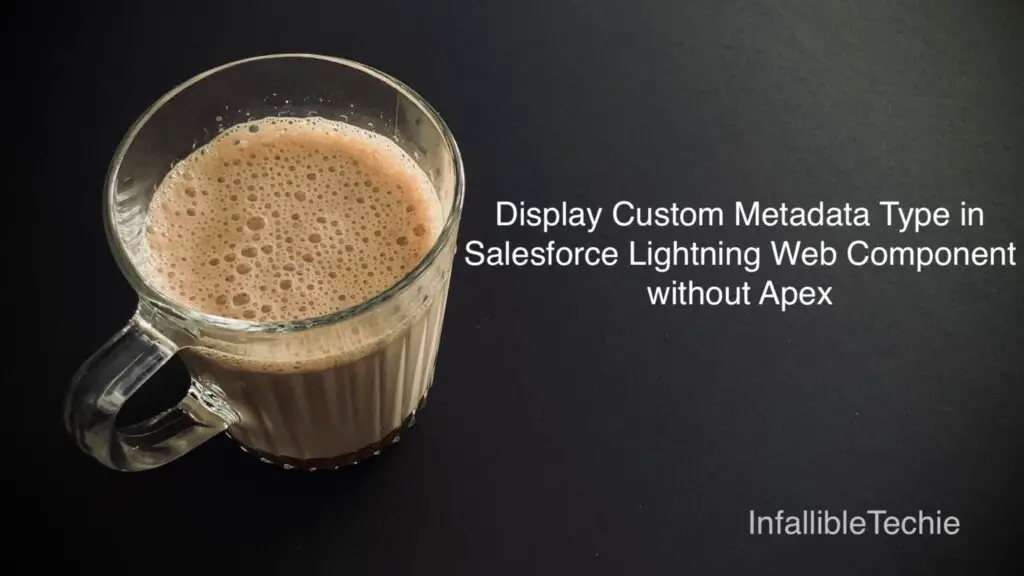
The GraphQL wire adapter can be used to fetch/retrieve and display Custom Metadata Type records in Salesforce Lightning Web Component without using Apex.
Sample Custom Metadata Type:
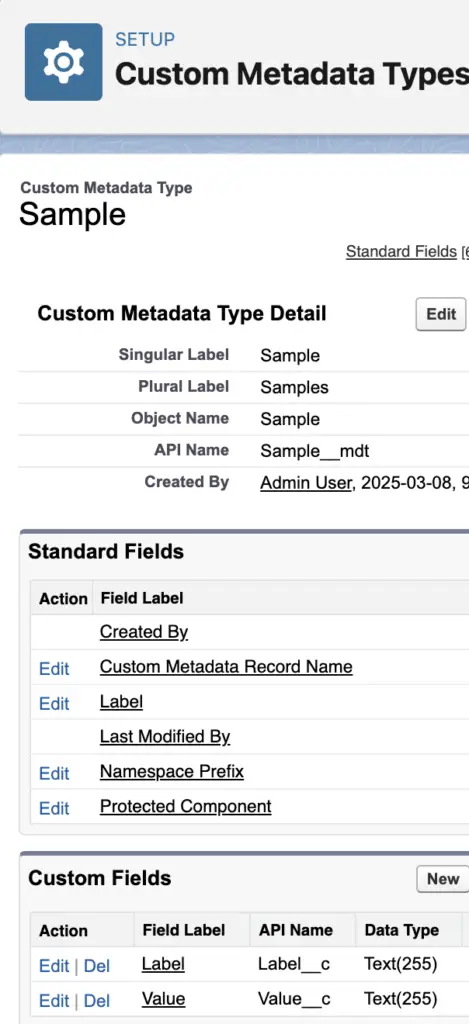
Sample Lighting Web Component:
HTML:
<template>
<lightning-card>
<div class="slds-m-around_medium">
<div style="width: 250px;">
<lightning-combobox
name="Sample"
label="Sample"
value={value}
placeholder="Select Sample"
options={options}
onchange={handleChange}>
</lightning-combobox>
<br/>
</div>
<template if:true={value}>
<p>Selected Value: {value}</p>
</template>
</div>
</lightning-card>
</template>
JavaScript:
import { LightningElement, wire } from 'lwc';
import { gql, graphql } from 'lightning/uiGraphQLApi';
export default class SampleLightningWebComponent extends LightningElement {
value;
@wire( graphql, {
query: gql`
query getSamples {
uiapi {
query {
Sample__mdt {
edges {
node {
Label__c : Label__c {
value
}
Value__c : Value__c {
value
}
}
}
}
}
}
}
`
})
graphql;
get options() {
console.log( this.graphql );
return this.graphql.data?.uiapi.query.Sample__mdt.edges.map(
( edge ) => ( {
label: edge.node.Label__c.value,
value: edge.node.Value__c.value
}
) );
}
handleChange( event ) {
this.value = event.target.value;
}
}
js-meta.xml:
<?xml version="1.0" encoding="UTF-8"?>
<LightningComponentBundle xmlns="http://soap.sforce.com/2006/04/metadata">
<apiVersion>63.0</apiVersion>
<isExposed>true</isExposed>
<targets>
<target>lightning__Tab</target>
</targets>
</LightningComponentBundle>
Output:
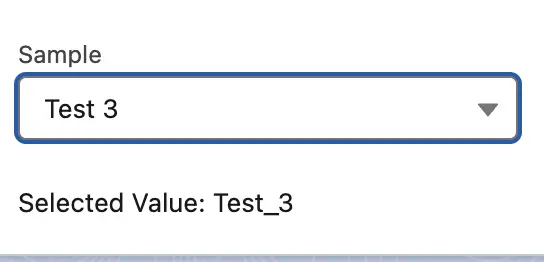