In this Blog Post, I have accessed the Google Gemini API from Salesforce Apex using the API Key approach.
Sample Apex Code:
public class GeminiAPIController {
public static String invokePrompt( String strMessage ) {
// Setting default value to return
String strResponse = 'Some issue with the API. Please try again later.';
// End Point of the API
String strEndpoint = 'https://generativelanguage.googleapis.com/v1beta/models/gemini-2.0-flash:generateContent?key=<YOUR API KEY>';
HTTP h = new HTTP();
HTTPRequest req = new HTTPRequest();
req.setEndPoint(
strEndpoint
);
req.setMethod(
'POST'
);
req.setHeader(
'Content-Type',
'application/json'
);
req.setBody(
'{ "contents": [ { "parts":[ {"text": "' +
strMessage + '" } ] } ] }'
);
HTTPResponse response = h.send(
req
);
System.debug(
'Response is ' +
response.getBody()
);
GeminiAPIWrapper objWrap = ( GeminiAPIWrapper )System.JSON.deserialize(
response.getBody(),
GeminiAPIWrapper.class
);
if ( objWrap.candidates.size() > 0 ) {
for ( GeminiAPICandidateWrapper objCaWrap : objWrap.candidates ) {
GeminiAPIContentWrapper objCoWrap = objCaWrap.content;
if ( objCoWrap != null ) {
if ( objCoWrap.parts.size() > 0 ) {
for ( GeminiAPIPartWrapper objPWrap : objCoWrap.parts ) {
System.debug( 'Text: ' + objPWrap.text );
// Retrieving the response for the Prompt
strResponse = objPWrap.text;
}
}
}
}
}
return strResponse;
}
// Wrapper Classes to handle the API Response
public class GeminiAPIWrapper {
List < GeminiAPICandidateWrapper > candidates;
}
public class GeminiAPICandidateWrapper {
GeminiAPIContentWrapper content;
}
public class GeminiAPIContentWrapper {
List < GeminiAPIPartWrapper > parts;
}
public class GeminiAPIPartWrapper {
String text;
}
}
Please add the Gemini API Endpoint URL in the Salesforce Remote Site Settings so that the HTTP Request to it will succeed. Make sure the Remote Site Setting is Active.
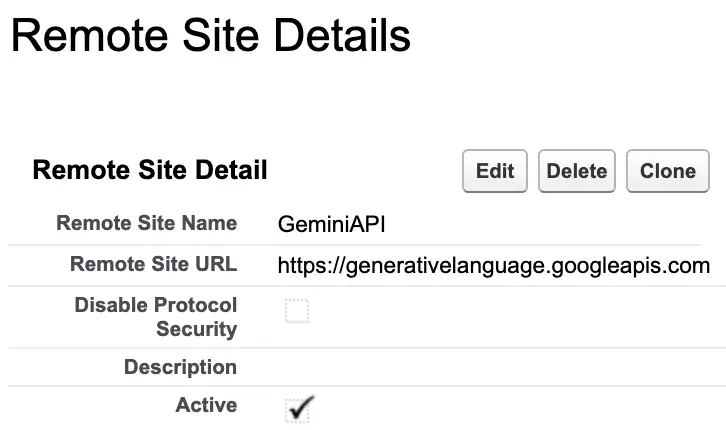