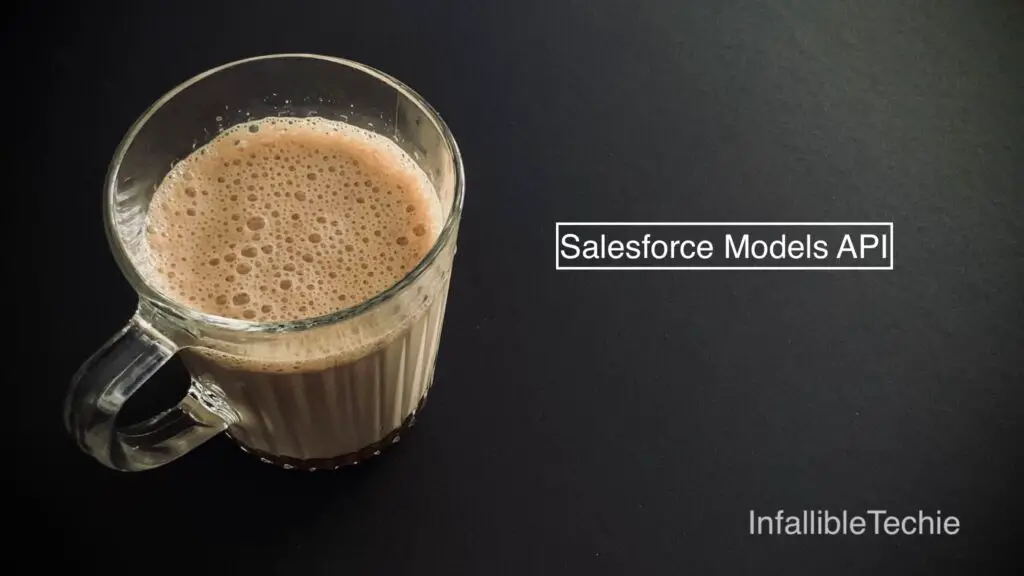
Salesforce Models API provides Apex classes and REST endpoints that can be used in your application to interact with the large language models (LLMs) from the Salesforce partners, including Anthropic, Google, and OpenAI.
All the requests made to the Salesforce Models API go through the Einstein Trust Layer which provides more security to the LLM requests.
Potential Use Cases:
Generate Text:
We can send a prompt to the Model and get the response back.
Generate Chat:
We can send single or multiple messages(whole Chat Conversation) with role(User or Agent or Assistant) to the Model and get the response. It helps us to generate the chat content with conversation history.
Generate Embedding:
We can generate embeddings using the Salesforce Model API. Embeddings are the numerical representations of the real-world objects that the machine learning (ML) and artificial intelligence (AI) systems or applications use to understand them.
Using the following Apex Code, I have invoked “OpenAI GPT 4 Omni (GPT-4o)” Model for a prompt request.
Sample Apex Code:
// Creating generate text request
aiplatform.ModelsAPI.createGenerations_Request request =
new aiplatform.ModelsAPI.createGenerations_Request();
// Specifying model as OpenAI GPT 4 Omni (GPT-4o)
request.modelName = 'sfdc_ai__DefaultGPT4Omni';
// Create request body
aiplatform.ModelsAPI_GenerationRequest requestBody =
new aiplatform.ModelsAPI_GenerationRequest();
request.body = requestBody;
// Add prompt to body
requestBody.prompt =
'What are the best restaurants in Toronto, Ontario, Canada';
try {
// Invoking the Models API
aiplatform.ModelsAPI modelsAPI = new aiplatform.ModelsAPI();
aiplatform.ModelsAPI.createGenerations_Response response =
modelsAPI.createGenerations(request);
System.debug(
'Models API response: ' +
response.Code200.generation.generatedText
);
// Handling Exception
} catch( aiplatform.ModelsAPI.createGenerations_ResponseException e ) {
System.debug(
'Response code: ' +
e.responseCode
);
System.debug(
'The following exception occurred: ' + e
);
}
Please check the following for the supported Models.
https://developer.salesforce.com/docs/einstein/genai/guide/supported-models.html
We can invoke the Models API via REST API too. Check the following steps for invoking Models API to use “OpenAI GPT 4 Omni (GPT-4o)” Model for a prompt request.
Steps for REST API invocation of Salesforce Models API:
1. Create a Connected App. Please complete steps 1.a to 1.f from the following blog post.
2. Get the Access Token. Use the above blog post for generating the Access Token.
3. Make a POST Request for invoking the Models API.
Endpoint:
https://api.salesforce.com/einstein/platform/v1/models/sfdc_ai__DefaultOpenAIGPT4OmniMini/generations
Headers:
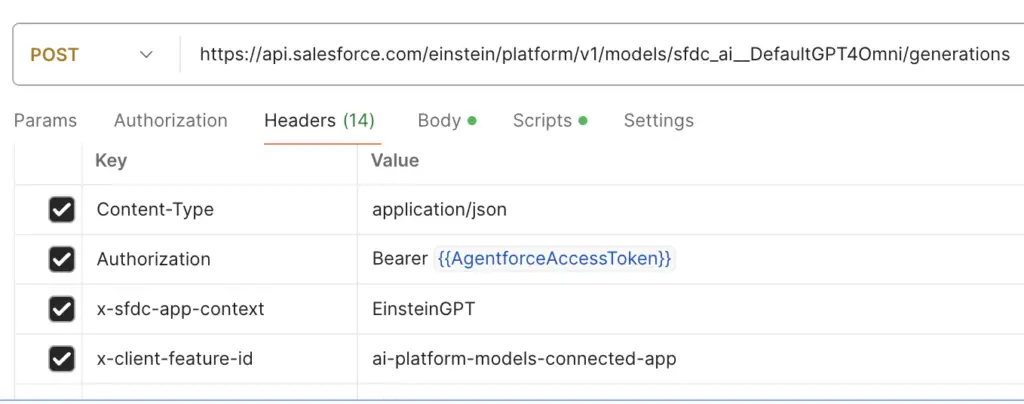
Body:
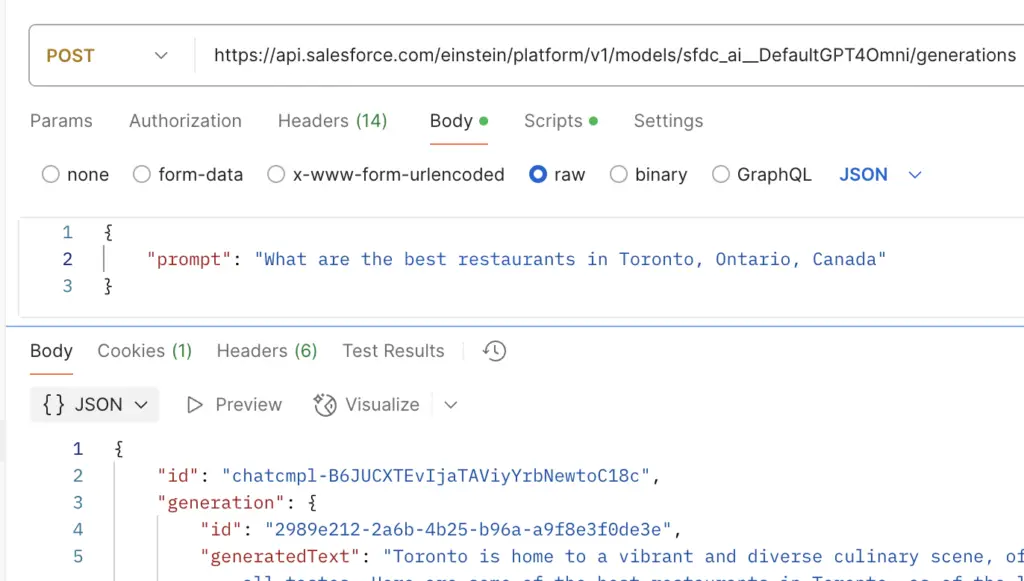
You can check the score for toxicity and content quality. The scores are available in the Data Cloud for reviewing them if needed. Score ranges from 0 to 1. If the score is higher, then the toxicity is higher.
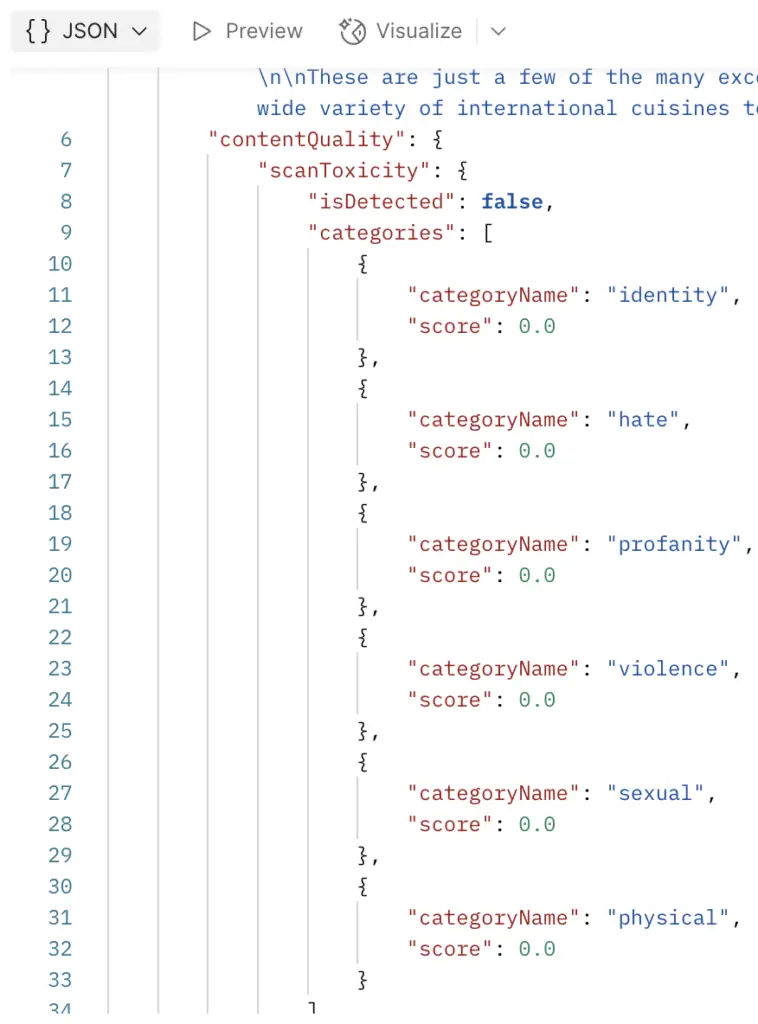
To know more about toxicity, please check the following:
https://developer.salesforce.com/docs/einstein/genai/guide/toxicity-scoring.html